One of the really easy ways to quickly define
your data model classes is to open up the Server Explorer tab within VS 2005
and connect to your database. You can then drill into the Tables and
Views within your database and drag/drop them from the server explorer onto the
DLINQ designer surface to automatically create data models for them. For
example, if I dragged/dropped the Suppliers, Customers, Orders, Order Details,
and Products tables onto the designer surface, by default I
would get a data model that looks like this:
Figure 4
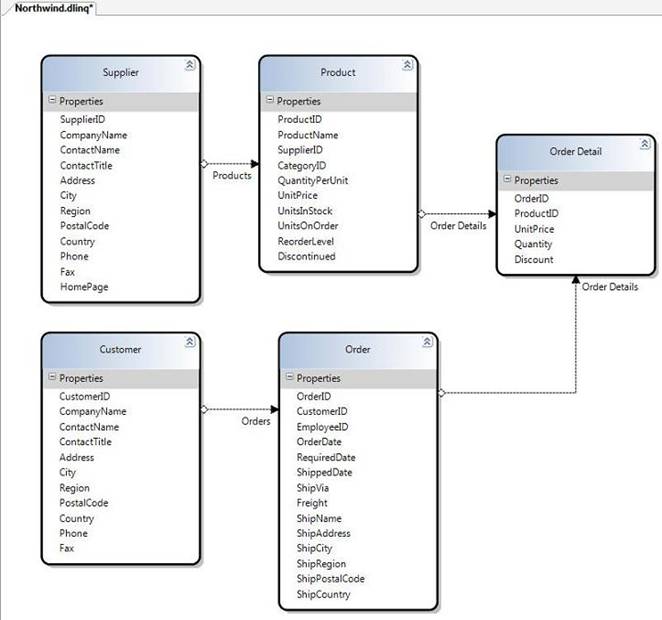
Note how the DLINQ designer automatically sets
up default associations between the different entities based on the Primary
Key/Foreign Key relationships in the database. These will automatically
cause properties to be created on the various data classes so that we can
perform rich querying and/or easily traverse between them. For example,
if I had a "Customer" instance above I could simply access the
"Customer.Orders" property to get a collection of all of the Customer's orders in the database.
If you want, you can add, delete or alter
these associations by simply clicking on the association links in the designer
and then use the property grid to change its settings. For example, if I
wanted to modify the "Order Details" associations above to be named
"OrderDetails," I would click on the association
line in the designer and change it via the property grid below. Or
because it supports inline editing for the name, I could also just click on the
name in the designer and rename it in place. See Figure 5 below for both
options.
Figure 5
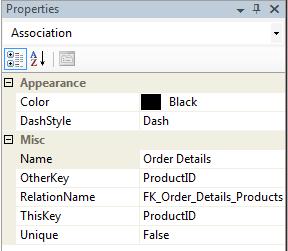
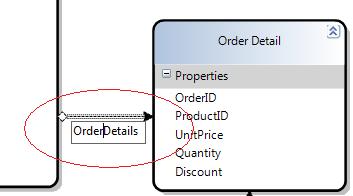
Additionally, I could use the designer to
easily rename the entities and/or rename/remove/add properties to them.
Once we are done defining your data models, we will just
save the file. The designer will then automatically generate a .cs or .vb
file containing the LINQ data class definitions. This is saved in a
nested file underneath the Northwind.dlinq item in the solution explorer.
Figure 6
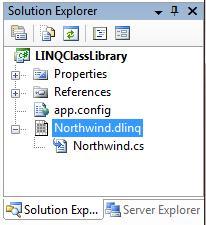
The LINQ data model classes created are
defined as "partial" classes" which means
we will be able to go in later and add entity and property validation rules
that enforce our business logic, as well as add any additional properties or
helper methods we want to the classes.