XAML attributes are equivalent to .NET class properties.
You can define attributes inline or explicit. Explicit definitions help you
when your element has several attributes and is getting larger.
Listing 4 shows an inline attribute definition for a Button's
Margin attribute and Listing 5 shows an explicit attribute definition for same
attribute.
Listing 4
<Button
Margin="5">
Click me!
</Button>
Listing 5
<Button>
<Button.Margin>5</Button.Margin>
Click me!
</Button>
Object oriented nature of XAML leads to a behavior that you
have seen in HTML before. Each attribute (which is actually a class property)
inherits its parent elements properties or overrides them (if you set that
property).
Another key topic in XAML attributes that you should know is
Attached Properties. Some XAML elements get their attributes in other elements
and these attributes are named Attached Properties.
For example when you want to use a Grid element (which is
very similar to Table in HTML) to lay out your elements, to specify the
position of elements in Grid's rows and elements you use Attached Properties.
Each element can get a Grid.Row and Grid.Column which represent the row and
column indexes for that element in Grid control. Listing 6 shows this in action
and you can see the output in Figure 2.
Listing 6
<Window x:Class="IntroductionToXAML.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="" Height="94" Width="108"
>
<Grid ShowGridLines="True">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="50" />
<ColumnDefinition Width="50" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="30" />
<RowDefinition Height="30" />
</Grid.RowDefinitions>
<Label Grid.Column="0" Grid.Row="0">0,0</Label>
<Label Grid.Column="0" Grid.Row="1">0,1</Label>
<Label Grid.Column="1" Grid.Row="0">1,0</Label>
<Label Grid.Column="1" Grid.Row="1">1,1</Label>
</Grid>
</Window>
Figure 2
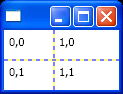