The code below explains when an instance constructor gets
called in class.
Listing 1
using System;
public class A
{
//Constructor of Class A
public A()
{
Console.WriteLine("Constructor of Class A");
}
}
public class B: A
{
//Constructor of Class B
public B()
{
Console.WriteLine("Constructor of Class B");
}
}
public class C: B
{
//Constructor of Class C
public C()
{
Console.WriteLine("Constructor of Class C");
}
}
public class Client
{
static void Main(string[]args)
{
// Initializing the class C's constructor.
C c = new C();
Console.Read();
}
}
Class A is the base class which has a child class B. Class C
gets inherited from Class B. All these classes have public instance
constructors. We create an object of Class C. Let us see the output once we
create an object of Class C.
Figure 1
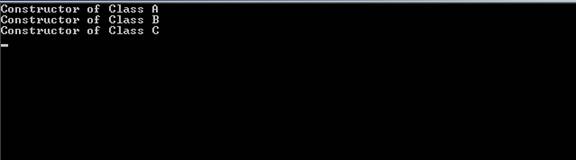
As shown in Figure 1, constructor of Class A gets called
first, constructor of Class B gets called and constructor of Class C then.
Class A is the base class and hence we understand that constructor of A gets
called first. This behavior of the code is expected. Now let us see a code
snippet where static constructors are also involved.
The code below exposes the difference between the instance
and normal constructors getting called.
Listing 2
using System;
public class A
{
//Static Constructor of Class A
static A()
{
Console.WriteLine("Static Constructor of Class A");
}
//Constructor of Class A
public A()
{
Console.WriteLine("Constructor of Class A");
}
}
public class B: A
{
//Static Constructor of Class B
static B()
{
Console.WriteLine("Static Constructor of Class B");
}
//Constructor of Class B
public B()
{
Console.WriteLine("Constructor of Class B");
}
}
public class C: B
{
//Static Constructor of Class A
static C()
{
Console.WriteLine("Static Constructor of Class C");
}
//Constructor of Class C
public C()
{
Console.WriteLine("Constructor of Class C");
}
}
public class Client
{
static void Main(string[]args)
{
// Initializing the class C's constructor.
C c = new C();
Console.Read();
}
}
Let us have a look at the output of the code snippet.
Figure 2
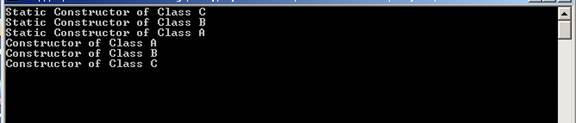
Surprised to see the output! I guess this is not the
expected behavior of the code snippet. Before understanding the difference
between the static and instance constructors we need to understand when does
static constructor gets called and when does instance constructor gets called.
A static constructor gets instantiated whenever the class is loaded for the
first time. Let us understand in detail what has happened.
When the .NET runtime executes the statement "new
C()" it understands that it has to create an object of C. Then it calls
the static constructor of class C. It then calls the static constructor of the
class B and then A. The instance constructor of the class A, B and C are then
called.