In order to create your own IWizard class, you need to follow
these steps:
·
Create a Project of the type Class Library.
·
By Default, the project will open a class file with the name
Class1. Change the class name appropriately.
·
You will need to import the following:
Listing 4
Imports System
Imports system.collections.Generic
Imports Microsoft.VisualStudio.TemplateWizard
Imports System.Windows.Forms
·
Make sure your class implements IWizard interface.
·
As soon as you implement the IWizard interface, VS will add the
methods that can be implemented in this class.
With the above
steps you just created your own Wizard implementation class. In our example we
are going to use a Window form to get user input regarding Server, database,
userID and password information. In order to create the window you will need
to follow these steps.
·
Right Click on the project and click on Add->Windows Form. With
VS 2005 you can also add dialog form directly by Add->New Item and selecting
Dialog template if you are interested.
·
The form will be added. Add four label controls and set the text
to Server:, Database:, UserID: and Password:. Add four textboxes and set the
ID property to txtServer, txtDatabase, txtUserID and txtPassword. Your form
should look like the following figure.
Figure 2
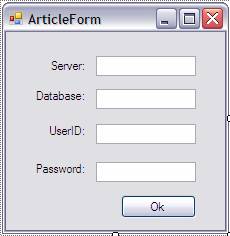
·
View Code for this form.
·
Declare a private variable for the LocalHostString to store the
connection String.
Private LocalHostString As String
·
Code the Ok buttons click event as follows.
Listing 5
Private Sub btnOk_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOk.Click
If txtServer.Text <> "" AndAlso txtDatabase.Text <> "" Then
Shell( _
"C:\Windows\system32\cmd.exe /C C:\WINDOWS\Microsoft.Net\Framework\v2.0.50727\aspnet_regsql.exe -S""" & _
txtServer.Text & """ -E -d""" & _
txtDatabase.Text & """ -A all", AppWinStyle.Hide)
LocalHostString = "Initial Catalog=" & txtDatabase.Text & ";Data Source=" &
txtServer.Text & ";User ID=" & txtUserId.Text &
";Password=" & txtPassword.Text
End If
Me.DialogResult = System.Windows.Forms.DialogResult.OK
Me.Close()
End Sub
·
In the above code we first do the registration using the Shell
command to call the ASPNet_regsql command and pass in the server and database
name. Once that is done, we build the LocalHostString based on the user input.
·
Now we need to create another function that will return the
LocalHostString.
Listing 6
Public Function GetLocalHostString() As String
Return LocalHostString
End Function
·
Now our form is ready to accept user input for server, database,
userID and password. The next step is to call this form through Wizard
implementation class.
·
In order to call this form, we will be implementing the
RunStarted method in our class.
·
We need to declare LocalHostString as Private variable on our
Implementation class too. Also, we will define the form as a private member.
Listing 7
Private dbform As ArticleForm
Private LocalHostString As String
·
This method will open the form, get the LocalHostString value and
then use the System.Collections.Generic.Dictionary to replace the $LocalHostString$
variable in our template. The code in RunStarted will look like Listing 8.
Listing 8
Public Sub RunStarted(ByVal automationObject As Object,
ByVal replacementsDictionary As System.Collections.Generic.Dictionary(Of String, String), _
ByVal runKind As Microsoft.VisualStudio.TemplateWizard.WizardRunKind, ByVal customParams() As Object) _
Implements Microsoft.VisualStudio.TemplateWizard.IWizard.RunStarted
dbform = New ArticleForm
dbform.ShowDialog()
LocalHostString = dbform.GetLocalHostString
replacementsDictionary.Add("$LocalHostString$", LocalHostString)
End Sub
·
In the above code we are opening the ArticleForm (my form) as a dialog
box and once the dialog box is closed we are getting the LocalHostString value.
We then add this variable to the dictionary to be used in our template at a
later stage.
·
Once this is created, you need to sign this assembly and then
install it in GAC to be used with your template.