In .NET, the threading is handled through the System.Threading namespace.
Creating a variable of the System.Threading.Thread
type allows you to create a new thread to start working with.
It is clear to everybody that the concept of threading is to
go off and do another task. The Thread constructor requires the address of the
procedure that will do the work for the thread.
The AddressOf is the parameter that the constructor needs to
begin using the thread.
Below is an example of a simple threading application.
Listing 1
Imports System.Threading
Public Class Form1
Inherits System.Windows.Forms.Form
Dim th1 As Thread
Dim th2 As Thread
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
th1 = New Thread(AddressOf proc1)
th2 = New Thread(AddressOf proc2)
th1.Start()
th2.Start()
End Sub
Sub proc1()
Dim iCount As Integer
For iCount = 1 To 10
cmb1.Items.Add(iCount)
Next
End Sub
Sub proc2()
Dim iCount As Integer
For iCount = 11 To 20
cmb2.Items.Add(iCount)
Next
End Sub
End Class
The output of the above code is shown in Figures 3 and 4. The
complete source code for our sample application can be downloaded from the
Downloads section given at the end of this article. The given code populates
two dropdowns on a form. Now let us see a comparison between the single
threaded application and the multithreaded application.
In the given scenario, if we implement the single thread
[demon thread], then we need to populate the 1st dropdown for which we need to
run a loop. After the 1st loop is over then go for the second loop.
Figure 3
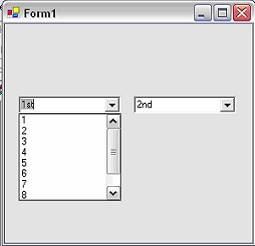
Figure 4
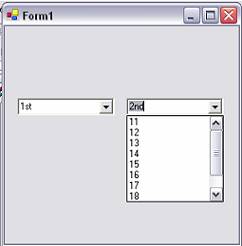
Here, in a multithreaded application, in order to populate
the 2nd dropdown, we do not need to wait for the population of the 1st one. That
means at the same time we can populate the two dropdowns; this bring the
execution time to half of the execution time of 1st one.
The above example is a simple one that demonstrates how the
multithreaded application can bring the execution time to (1/n)th of the total
execution time, where n = Number of threads used.