Now that you have the foundation in place to utilize the XML tags recommended by Microsoft, let us now take a look at the SQLServerDAL class that is part of Microsoft .NET Petshop 3.0. The reason I have selected this class is because Microsoft has done a great job of documenting the ExecuteReader method.
Listing 1: C# Class Example XML Documentation
namespace PetShop.SQLServerDAL {
/// <summary>
/// The SqlHelper class is intended to encapsulate high performance,
/// scalable best practices for common uses of SqlClient.
/// </summary>
public abstract class SQLHelper {
//Database connection strings
public static readonly string CONN_STRING_NON_DTC =
ConnectionInfo.DecryptDBConnectionString(ConfigurationSettings.AppSettings["SQLConnString1"]);
public static readonly string CONN_STRING_DTC_INV =
ConnectionInfo.DecryptDBConnectionString(ConfigurationSettings.AppSettings["SQLConnString2"]);
public static readonly string CONN_STRING_DTC_ORDERS =
ConnectionInfo.DecryptDBConnectionString(ConfigurationSettings.AppSettings["SQLConnString3"]);
/// <summary>
/// Execute a SqlCommand that returns a resultset against the database specified in the connection string
/// using the provided parameters.
/// </summary>
/// <remarks>
/// e.g.:
/// SqlDataReader r = ExecuteReader(connString, CommandType.StoredProcedure, "PublishOrders",
/// new SqlParameter("@prodid", 24));
/// </remarks>
/// <param name="connectionString">a valid connection string for a SqlConnection</param>
/// <param name="commandType">the CommandType (stored procedure, text, etc.)</param>
/// <param name="commandText">the stored procedure name or T-SQL command</param>
/// <param name="commandParameters">an array of SqlParamters used to execute the command</param>
/// <returns>A SqlDataReader containing the results</returns>
public static SqlDataReader ExecuteReader(string connString, CommandType cmdType, string cmdText,
params SqlParameter[] cmdParms) {
SqlCommand cmd = new SqlCommand();
SqlConnection conn = new SqlConnection(connString);
// we use a try/catch here because if the method throws an exception we want to
// close the connection throw code, because no datareader will exist, hence the
// commandBehaviour.CloseConnection will not work
try {
PrepareCommand(cmd, conn, null, cmdType, cmdText, cmdParms);
SqlDataReader rdr = cmd.ExecuteReader(CommandBehavior.CloseConnection);
cmd.Parameters.Clear();
return rdr;
}catch {
conn.Close();
throw;
}
}
}
As you can see by the example code in Listing 1, the documentation comments for the ExecuteReader method have made use of the <summary>, <remarks>, <param>, and <returns> tags.
At this point you may be asking yourself, “What if I have written this class in VB.NET?” While it is true that in Visual Studio .NET XML documentation is supported in C# but not in VB.NET, there is a solution to overcome this obstacle: take a look at the VBCommenter PowerToy Development workspace. By utilizing this free plug-in, there is absolutely no reason why any developer who uses VB.NET should ever say, “I would document my code, however Microsoft has not provided the ability for me to do so.” To learn more about using this plug-in, read the MSDN article, Creating Documentation for Your Visual Basic .NET Application.
Figure 2: Generated Documentation From VB.NET Code
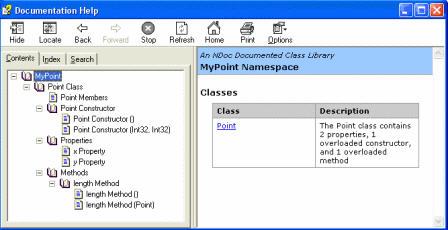
Credit: The above image is from the article Creating Documentation for Your VB .NET Application.
Figure 3: NDoc Documentation from C# Code
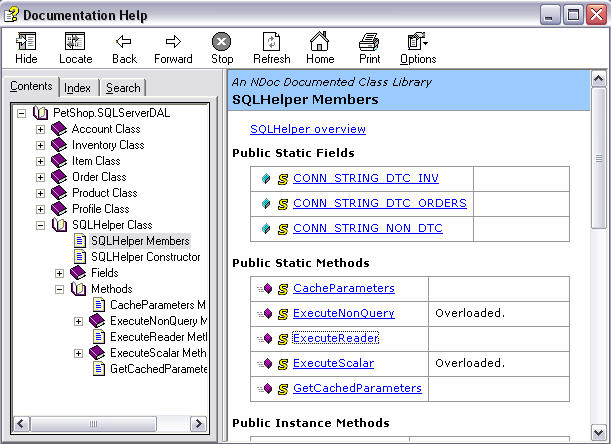
Credit: The above image was generated from the SQLServerDAL class using NDoc.
Following are five points that I personally feel should be taken into consideration when you begin to develop:
- Comments must add to the clarity of your code. The reason you document your code is to make it more understandable to you, your coworkers, and any other developer who comes after you.
- If your program is not worth documenting, it probably is not worth running.
- Avoid decoration, i.e., do not use banner-like comments.
- Keep comments simple. Some of the best comments I personally have ever seen are simple and straightforward.
- Write the documentation before you write the code. This gives you an opportunity to think about how the code will work before you write it and will ensure that the documentation is completed. But you should at least document your code as you write it.
At this point I have discussed the recommended XML document tags, provided a sample documented class, and presented the compiled help file. I hope that you agree that documentation is definitely a powerful and important process.